PHP: A Step by Step Introduction To Programming in PHP
Author: Phil Askor
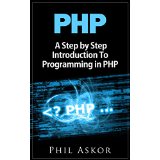
Book Series: Software Guides
I. Introduction
What is PHP?
Did you know that PHP is used by over 81% of all websites? PHP is used by many high traffic websites, including Facebook, YouTube and Amazon. Rasmus Lerdorf originally created PHP in 1994 in an effort to keep track of the number of visitors who viewed his online resume. PHP was not intended as a programming language, but as what could be considered as an early version of an analytic tool. Lerdorf named the scripts “Personal Home Page Tools”, which would later be abbreviated as PHP Tools. Lerdorf made the scripts public in 1995 after rewriting them and adding more functionalities, such as the possibility to create a guest book so that visitors could leave a message on his website.
A new version of the code was released in April 1996. This new generation featured an HTML-embedded syntax, automatic interpretation of form variables, and built-in support for a number of databases. PHP has successfully evolved from a collection of web page construction tools into a programming language. This early version of PHP was used by over 60,000 websites in 1998, which represented about 1% of the Internet at the time.
The third generation of PHP was announced in June 1998. The language was renamed PHP, an acronym that stands for Hypertext Preprocessor. PHP 3.0 features a more consistent syntax, an improved interface, as well as compatibility with a greater number of databases. PHP 3.0 attracted many developers thanks to its potential for growth. PHP 3.0 was installed on over 10% of Internet servers in 1998.
PHP is currently in its sixth generation and a seventh generation has been announced. Developers are still working on improving the syntax for the new generation. PHP has come a long way, and is now used by over 240 million websites and 2.1 million web servers. The PHP Group is now in charge of performing tests, creating documentation, and improving the language.
PHP became so popular because it is easy for beginners to use, and offers endless possibilities to more advanced programmers. It is possible to start writing scripts after spending only a few hours studying PHP. Compared to other languages such as C or Perl, PHP requires only a few commands for a working script.
PHP basically “tells” a web server to create HTML according to a script. This allows for more dynamic and interactive web pages. PHP, for instance, used to allow users to post a message on a forum, sign up for a newsletter, place an item in a shopping cart, or give users the ability to register and log in to a site.
What Is PHP Used For?
PHP has many uses, including sending and receiving cookies, collecting data from a form, or creating dynamic content for a page. PHP is very flexible thanks to its compatibility with many output formats (including XML, PDF, and even Flash movies), databases, web servers, and web browsers. PHP usage can be organized in three main categories:
Creating scripts for a web server. This is the most common use for PHP. This requires a PHP server module (or Common Gateway Interface), a server, and a web browser. The web server hosts the PHP content and requires a PHP server module in order to properly interpret the PHP scripts stored on it. The web browser displays the output of the script.
PHP can be used to program a computer through a command line. A PHP parser is required, as well as an operating system compatible with PHP (a wide majority of operating systems are). PHP scripts can be used to create processing tasks, for instance through the Task Scheduler in Windows. This is a less common use of PHP.
Advanced PHP users can create desktop applications with this language. An extension called PHP-GTK is needed to create applications with PHP. This is a good way to create applications compatible with multiple platforms, but not always an ideal method for a pleasant graphical and user-friendly interface. This is the least common use for PHP.
Creating scripts that can be interpreted by a web browser is the most common use for PHP. This feature allows webmasters to create interactive content, such as a unit conversion tool, an automated translator, or taking an online quiz. The basic concept behind interactive scripts is that the input of the user influences the content that is generated by the server.
PHP can also be used to record content to a database. PHP is compatible with many databases, but MySQL is probably the most common one. Internet users can enter information in a form, and the PHP script will write or retrieve content from a database. Writing content in a database is a feature that allows users to register, log in, subscribe to a newsletter, or enter a contest. Retrieving content from a database can, for instance, allow a user to perform an advanced search on an online store and find relevant products.
How to Install PHP
Before uploading PHP scripts to a server, it is necessary to set up the server so it can process PHP requests. Apache servers, Microsoft Internet Information servers, iPlanet, Netscape, and many other servers have a Server Application Programming Interface (referred to as SAPI). This is a DLL file that functions as an interface between the server and PHP scripts. In other words, the DLL file interprets PHP scripts in a way that is understood by the server. If the DLL file is not already present on the server, it is necessary to upload it before the server can interpret PHP scripts.
If a server does not have an interface compatible with PHP, it is necessary to configure the server to use an executable interface. The installation process varies from one server to another. The server should be configured to support CGI (Common Gateway Interface), and a module such as FastCGI should be installed on the server. It is best to contact the person responsible for the server to get detailed instructions on how to install and configure PHP on a server that does not already have an interface.
How to Create a PHP File
There is no need to create a separate file for PHP scripts. PHP code can be integrated within a HTML page, and it is possible to jump in and out of PHP mode thanks to the delimiters “”. The delimiters can be used multiple times on a page to run different scripts. An HTML page could, for instance, have a PHP script designed to allow users to log in, and another script so that users can comment on an article. Integrating a PHP script into HTML code is very easy:
Step 1: Create a basic HTML page.
The header should contain a title, meta tags, a meta description, and perhaps a link to a CSS style sheet. The body should contain your main content. You can add a footer for your copyright or contact information.
Step 2: Add the PHP delimiters.
?>
Note that the PHP delimiters are placed within a paragraph, so the PHP script is displayed as a separate block. This also means the values set for paragraphs on a CSS style sheet will apply to this block of content. A CSS style sheet can, for instance, be used to define the font, size, or color of the text.
Step 3: Add a PHP script within the delimiters.
echo “Enter your text here”;
?>
In this example, the function “echo” is used to display a text. Note that the text should be contained within quotation marks, and that the line should end with a semi-colon.
Step 4: Save your file as a PHP file.
The file extension .php should be used when saving a file that contains PHP code. If the file extension .html is used, the PHP code will not be interpreted as such, and the PHP script will not work. A homepage file would, for instance, be called index.php instead of index.html.
A Brief Overview of PHP Syntax
PHP syntax is fairly simple, but it is important to memorize the different functions and their use. Note that a semi-colon is used at the end of every line.
Echo: the function of “echo” is to output a string of text.
echo “Enter your text here”;
?>
Strings: strings are sentences within quotes. A string is basically the content that will be displayed to users. Strings can be a single block of text like in the example “Enter your text here”, but it is also possible to link several strings together by placing a dot between them if they need to remain separated.
echo “Enter” . “your text” . “here”;
?>
Arithmetic: PHP can be used to do math. Enter operations using the symbols +, -, / or * and the result will be displayed.
echo 45 * 34;
?>
Variables: variables are used for more complex coding. A variable can be used to save a value such as a string or a number and assign a name to it. A variable always starts with a $, but the name of a variable can be anything.
$name = “John Smith”;
$emailaddress = “johnsmith@email.com”;
?>
Note that these variables are not displayed to visitors. In order to make a variable visible, echo should be added before it. A variable can, for instance, be defined at the beginning of a document and repeated multiple times by using echo.
$name = “John Smith”;
$emailaddress = “johnsmith@email.com”;
echo $name;
echo $emailaddress;
?>
Semi-colons and statements: semi-colons are used at the end of each statement. Statements are the shortest unit of code in PHP. Here are a few examples of statements:
echo “Enter your text here”;
echo 45 * 34;
$name = “John Smith”;
Comments: it is possible to add comments to PHP code. Comments are not displayed on web pages, they are there to help the web developer.
echo “Enter your text here”;
// Enter your comment here.
?>
II. PHP's Flow Control
It is possible to control the output of a PHP script by establishing certain conditions. Several conditions can be used to determine the outcome. Flow control can be used to compare values, display a specific string if a condition is met, and even choose in which order conditions are evaluated.
Comparisons
Flow control includes comparing strings. For instance, comparisons can be used to trigger a specific action if a value or a number is greater than another, or falls between two other values. These are the symbols used to make comparisons between elements:
> Greater than
< Smaller than
>= Greater or equal
<= Smaller or equal
== Equal
!= Not equal
True and False Conditions
PHP can be used to ask questions and display a specific string depending on the answer. The answer selected or entered determines the output thanks to if statements. If the condition is met and the answer is yes, the string will be displayed. The keyword “if” and curly brackets are used to specify what happens if the condition is met.
Creating a Condition
In this simple example, flow control is used to greet a user if their name is recognized.
Step 1: Set a variable for “if”.
if($name= “John Smith”)
?>
Step 2: Specify what happens if the condition is met. Note that the string should be placed within curly brackets, and the semi-colon is included in the brackets.
if($name= “John Smith”) {
echo “Welcome back John!”; }
?>
Step 3: Input a variable. For this example, the variable entered meets the requirement set by “if” for the string to be displayed.
$name= “John Smith”;
if($name= “John Smith”) {
echo “Welcome back John!”; }
?>
In this second example, flow control is used to display a string of text about benefiting from free shipping if the cost of an order is greater or equal to a specified value.
Step 1: Set a variable for the “if” condition.
if($cost >= 50)
?>
Step 2: Determine what happens if the condition is met.
if($cost >= 50) {
echo “You get free shipping on your order!”; }
?>
Step 3: Input a variable. For this example, the cost entered exceeds the value set in the first step, so the condition is fulfilled and the string is displayed.
$cost = 70;
if($cost >= 50) {
echo “You get free shipping on your order!”; }
?>
Using an “Else” if the Condition Is Not Fulfilled
Control flow makes it possible to select a default action in case the condition set by “if” is not fulfilled. Establishing a specific action for every possibility would take a lot of time and would not be useful. The keyword “else” can be used to set a default action, and the condition set by “if” becomes an exception. The keyword “else” requires a syntax that is very similar to the keyword “if” with curvy brackets and a semi-colon within the brackets.
In this example, “else” is used to display a default greeting to users, while a few users get a personalized greeting.
Step 1: Establish an exception with “if”.
if($name= “John Smith”) {
echo “Welcome back John!”; }
?>
Step 2: Add the “else” keyword.
if($name= “John Smith”) {
echo “Welcome back John!”; }
else {
?>
Step 3: Defines what happens if the condition is not met. Note that the string is within curly brackets, and the semi-colon is included in the curly brackets.
if($name= “John Smith”) {
echo “Welcome back John!”; }
else {
echo “Welcome guest!”; }
?>
Step 4: Set a variable for the condition. In this example, the variable entered does not fulfill the “if” condition so the action defined by “else” will occur.
$name= “Jane Doe”;
if($name= “John Smith”) {
echo “Welcome back John!”; }
else {
echo “Welcome guest!”; }
?>
In this second examples, “else” is used to display a default message if the user does not qualify for free shipping. The “if” condition is fulfilled if the value entered is equal or greater than a specific value.
Step 1: Determine what happens if the condition is fulfilled.
if($cost >= 50) {
echo =“You get free shipping on your order!”; }
?>
Step 2: Add the “else” keyword and the default action in case the “if” condition is not fulfilled.
if($cost >= 50) {
echo “You get free shipping on your order!”; }
else {
echo “Did you know you can benefit from free shipping on orders over $50?"; }
?>
Step 3: Input a value. For this example, the cost of the order is too low for the user to benefit from free shipping and the string associated with the “else” keyword will be displayed.
$cost = 30;
if($cost >= 50) {
echo “You get free shipping on your order!”; }
else {
echo “Did you know you can benefit from free shipping on orders over $50?"; }
?>
Switch Statements
Control flow can quickly become complex if there is a need for a chain of “if” or “else” keywords. A “switch” statement is used to simplify things and make PHP code more readable. The function of a “switch” statement is very similar to the one of the “if” keyword. A specific block of code is executed if a condition defined by the “switch” statement is fulfilled.
Creating a “switch” statement is built like an “if” statement. In this example, a “switch” statement is used to display a personalized greeting if a user's name is recognized. Every possibility or “case” is considered until one is fulfilled. If the variable does not correspond to any of the values defined by “cases”, the default statement is executed.
Step 1: Defining the variable that influences the outcome of the “switch” statement. Note that the syntax is very similar to what comes after “if”.
switch ($name)
?>
Step 2: List different possibilities and determine what happens for each of them. The keyword “case” is used to refer to each possibility. Note that colons are used after each case, and semi-colons are used at the end of the string once the outcome has been associated with the case. The “cases” are placed within curly brackets, and the variable for each “case” is placed within apostrophes. The keyword “break” is added after each statement to indicate they are separate entities.
switch ($name) {
case 'John Smith':
echo “Welcome back John!”;
break;
case 'Jane Doe':
echo “Welcome back Jane!”;
break; }
?>
Step 3: Add a default action if the variable does not correspond to any of the “cases”. The keyword “default” should be used and the syntax is similar to the “cases”.
switch ($name) {
case 'John Smith':
echo “Welcome back John!”;
break;
case 'Jane Doe':
echo “Welcome back Jane!”;
break;
default:
echo “Welcome guest!”;
}
?>
Step 4: Add a variable. In this case, the variable entered does not correspond to any of the “cases” and the default statement will be executed. The user will see the text “Welcome guest!” displayed.
$name = “Sam”;
switch ($name) {
case 'John Smith':
echo “Welcome back John!”;
break;
case 'Jane Doe':
echo “Welcome back Jane!”;
break;
default:
echo “Welcome guest!”;
}
?>
The keyword “break” separates the different possibilities and their outcomes. However, it is possible to remove it if there is a single desired outcome for more than one “case”. The “break” keyword disappears from the syntax and “cases” are listed before the statement that should be executed if any of these “cases” is fulfilled. Here is an example:
switch ($name) {
case 'John Smith':
case 'Jane Doe':
case 'Sam Carpenter':
echo “Welcome back!”;
break;
default:
echo “Welcome guest!”;
}
?>
III. Storing a Set of Values With Arrays
Working with large quantities of information can quickly become complex. Arrays are used to make things easier if there is a need to arrange information. Arrays are similar to variables, but they can contain more than one element. This is an efficient way to apply a single line of code to a group of elements instead of applying this line of code to multiple variables.
Array syntax is very similar to variable syntax. The name of an array is always preceded by $ and followed by =. They keyword “array” has to be used after the name of the array to indicate that this is an array and not a variable. The elements of the array are placed between parentheses and separated by commas. Each element should be placed between quotation marks, and the statement should, of course end, with a semi-colon.
In this example, an array is created so a line of code can be applied to a group of customers.
Step 1: Creating the array and indicating its function with the keyword “array”.
$customer = array
?>
Step 2: Add elements to the array.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
?>
It is possible to access a specific element in a given array. The elements placed within an array are numbered starting from 0. In the above example, the element “John Smith” is numbered 0, “Jane Doe” is numbered 1, and “Sam Carpenter” is numbered 2. Any of these elements can be accessed by using offsets. Offsets can be created with curly brackets or with square brackets.
In this example, an offset is used to display the name of a customer. Note that the number placed within the offset is 1, which corresponds to the element “Jane Doe” of the array $customer.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
echo $customer[1]
?>
Referring to a specific element in an array comes in handy if there is a need to modify or even remove an element from an array. An element can be modified by creating an offset and establishing a new value for this element. Here is an example where a customer's name is replaced by another value.
Step 1: Create an array and add an offset for the element that needs to be modified.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
$customer[1]
?>
Step 2: Establish a new value for the element selected in the offset. The new value should be placed within quotation marks.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
$customer[1] = “a valued customer”;
?>
Step 3: Now that the element has been modified, it is possible to display the new value by using an offset. In this example, the text “a valued customer” will be displayed instead of “Jane Doe”.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
$customer[1] = “a valued customer”;
echo $customer[1]
?>
Deleting elements from an array requires a different syntax than the one used to modify them. The keyword “unset” should be used to remove a specific element from an array. In this example, an element is removed from the array $customer.
Step 1: Create an array and add the “unset” keyword. The following syntax would cause the entire array to be deleted. The name of the array should be placed within parentheses.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
unset($customer);
?>
Step 2: Add an offset to indicate which element needs to be removed from the array.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
unset($customer[0]);
?>
IV. Repeating Code With Loops
Loops can be used to repeat a bit of code to make things easier. Loops make PHP code shorter and easier to create. Loops also allow for modifications to the code that is being repeated. Loops are ideal if there is a need for generating a list, or if an element needs to be repeated. There are different types of loops depending on which function is needed.
How to Create a “For” Loop
The purpose of a “for” loop is to repeat a specific bit of code. The “for” loop will repeat the code a certain number of times. This type of loop should be used when the webmaster knows how many times the bit of code needs to be repeated. If a factor, such as a variable entered by a user, influences the number of times the code should be repeated, another type of loop is needed.
The “for” loop syntax begins with the “for” keyword. The loop needs to include three different elements between parentheses: where the loops starts, where it ends, and what to do to find the next iteration of the loop. These three elements need to be separated by a semi-colons. A curly bracket should be opened next, to indicate the string of code that needs to be repeated.
In this example, a “for” loop is created to count from 0 to 20. Instead of creating a code that displays each number, the loop will calculate each element of the list.
Step 1: Create the “for” loop and determine when the loop starts.
for ($numbers = 0;
?>
Step 2: Determine where the loop ends and which step should be taken to generate the next iteration in the list.
for ($numbers = 0; $numbers <= 20; $numbers +)
?>
Step 3: Add the string of code that needs to be repeated between curly brackets. Note that ++ is used to add 1 to each iteration. $numbers= $numbers + 3 could be used to skip numbers.
for ( $numbers = 0; $numbers <= 20; $numbers ++ ) {
echo $numbers; }
?>
Infinite loops can occur if the second element of the loop is not properly set. It is important to make sure this second element is exact, or the loop will never end. Using $numbers + 1 as the third element of the loop instead of $numbers++ is another common mistake. Using $numbers + 1 will not work.
Creating a “Foreach” Loop
A “foreach” loop applies a bit of code to different elements. Instead of repeating multiple elements and applying the code to each, the elements can be placed in an array, and a “foreach” loop can be created so the code runs for each element of the array.
The syntax is similar to the “for” loop. The keyword “foreach” is used, and the array that needs to be put through a loop is placed within parentheses. Curly brackets are then used for the line of code that should be repeated.
In this example, an array that contains the name of different customers is redefined as a new variable and this new variable is displayed, thanks to a “foreach” loop.
Step 1: Create an array.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
?>
Step 2: Create a “foreach” loop and associate it with the array. Temporarily redefine the array by giving it a new value with the keyword “as”.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
foreach ($customer as $names)
?>
Step 3: Add the bit of code that will be applied to all the elements of the array within curly brackets.
$customer = array (“John Smith” , “Jane Doe” , “Sam Carpenter”);
foreach ($customer as $names) {
echo $names; }
?>
Creating a “While” Loop
A “while” loop is used if the number of iterations is unknown. For instance, a “while” loop could be used to generate random iterations and stop when a specific event happens. In other words, a “while” loop will be executed for as long as a certain condition is true.
The syntax of a “while” loop is similar to the syntax used for “for” and “foreach” loops. The keyword used is “while”, and a condition is placed within parentheses. A bit of code should be placed within curly brackets. This code will be executed as long as the condition within the parentheses is true.
It is very important to avoid infinite loops. A “while” loop should always include a variable that defines when the loop stops.
In this example, a “while” loop is created to generate numbers. The loop will generate new iterations by adding 2 to the previous iteration and stop once 20 is reached.
Step 1: Creating a “while” loop. The keyword “while” is used and parentheses are used for the condition that needs to be fulfilled for the loop to stop.
while ( )
?>
Step 2: Determining when the loop starts and when it stops. In this example, the loop will start with the number 2 and stops once the number 20 is generated by the loop.
$number = 2;
while ($number <= 20)
?>
Step 3: The line of code that is to be repeated by the “while” loop is added between curly brackets, along with information on how to generate a new iteration of the value.
$number = 2;
while ($number <= 20) {
echo “generated number: {$number}”;
$number +2;
?>
Creating a “Do”/”While” Loop
A “while” loop will keep checking if the condition is fulfilled before a new iteration of the code is issued. A “do”/”while” loop will check if the condition is fulfilled after each iteration is issued. This means the code of a “while” loop has to be executed at least once (and will stop if the condition is fulfilled). A “do”/”while” loop can be used to bypass a bit of code if the condition is already fulfilled.
The syntax of a “do”/”while” loop is similar to the “while” loop, except the condition is checked after the first iteration. The loop should begin with a statement that establishes a value for a variable. The keyword “do” is then used along with the line of code that should be executed within curly brackets. The keyword “while” is added after the “do” portion of the loop, and the condition for the loop to keep running is added within parentheses.
In this example, a “do”/”while” loop is created to run only once, since the condition for the loop to keep running is not fulfilled. A number is assigned to a variable (3) and the loop is set to keep running until the value is greater than 3. The condition is not fulfilled, and the loop will stop running after issuing one iteration.
Step 1: Creating a variable and assigning a value to it.
$number = 3;
?>
Step 2: Adding the “do” keyword and the string of code that will be executed by the loop. The code is placed within curly brackets. In this case, the number is displayed thanks to “echo”.
$number = 3;
do {
echo $number; }
?>
Step 3: Add the “while” keyword to define what will cause the loop to stop. In this case, the condition associated with while is false, and the loop will stop after only one iteration.
$number = 3;
do {
echo $number; }
while ($number > 3);
?>
Generating Random Values With a “Do”/”While” Loop
Loops can be used to generate random numbers and stop once a specific event happens. For instance, a loop could generate values until a specific value is iterated, or until a value is iterated a specific number of times in a row. In this example, a “while” loop is created to simulate a rolling dice. The loop will stop once a 6 is randomly generated.
Step 1: Set a starting point for the “do”/”while” loop. In this case, the starting point is the variable $dice (representing the number of times the dice has been rolled), and the value associated with it is 0.
$dice = 0;
?>
Step 2: Create the “do” portion of the loop, and place the code that is to be repeated within curly brackets. In this case, the code consists of displaying a random number between 1 and 6 to simulate a dice being cast. A new variable is introduced to represent the outcome of the dice being cast. The keyword “rand” is used to generate a random number.
$dice = 0;
do { $outcome = rand (1,6);
?>
Step 3: Add another element to the “do” portion of the loop so the loop keeps track of how many times the dice has been rolled. With every iteration of the code, one is added to the variable $dice. “Echo” is also added to display the outcome of the dice being rolled.
$dice = 0;
do { $outcome = rand (1,6);
$dice ++;
echo $outcome; }
?>
Step 4: Add the “while” keyword and the condition that will cause the loop to keep running. In this case, the loop should keep running until a 6 is randomly generated. The loop should keep running as long as the variable $number is not equal to 6.
$dice = 0;
do { $outcome = rand (1,6);
$dice ++;
echo $outcome; }
while ($dice !=6);
?>
Step 5: Add a finishing touch by using “echo” and the variable $dice to let the user know how many times the dice was rolled until they got a 6.
$dice = 0;
do { $outcome = rand (1,6);
$dice ++;
echo $outcome; }
while ($dice !=6);
echo “You got a 6 in {$dice} tries!”;
?>
V. Using Functions to Perform Actions
Functions allow programmers to perform actions. Some functions are already built into PHP (for instance, the function “round” is used to round decimal numbers). The programmer can define other functions. The possibilities are endless, since there are many functions built into PHP, and additional functions can be created as needed!
Functions are bits of code that can be used to manipulate strings, numbers, or arrays. Functions can be referred to throughout the code once they have been established, to avoid repetition. In the following example, a simple function is used to calculate the number of characters in a sentence.
Step 1: Create a variable so the function can be associated with it. The function “strlen” is a built-in function that calculates the number of characters in a word or a sentence.
php
$characters = strlen
?>
Step 2: Add the word or the sentence in parentheses after the function. Add echo followed by the variable created in step 1 so the number of characters can be displayed.
php
$characters = strlen (“Enter your sentence here”);
echo $characters;
?>
Step 3: In step 2, a string of text was directly associated with the function. However, it is possible to run a variable through a function to avoid repetition. The string of text is replaced by a variable, and the variable is defined before the function.
$sentence = “Enter your sentence here”;
$characters = strlen (“Enter your sentence here”);
echo $characters;
?>
How to Create a String Function
String functions are applied to a string of text. It is possible to retrieve a specific piece of a text string, display a text in uppercase letters, or transform an uppercase string of text into a lowercase text. These built-in functions are very easy to use and are often used by webmasters to format text.
In the following example, the function “substr” is used to extract a portion of text referred to as substring. The substring is defined by numbers that refer to the characters that should be extracted from the original string of text.
Step 1: Create a variable and assign a value. This is the original string of text.
$text = “Enter your text here”;
?>
Step 2: Create a new variable, add the “substr” function and define its characteristics between parentheses. The first element placed between parentheses is the variable created in step 1. The second element indicates the first character that should be displayed, and the third element indicates which character should be displayed last. Note that commas separate these elements.
$text = “Enter your text here”;
$substring = substr($text, 0, 7);
?>
Step 3: It is now possible to refer to the “substr” function created in step 2 to display parts of the value assigned to the variable $ text. “Echo” is used to display the variable $substring created in step 2. Since the characteristics of the function were 0 and 7, the first seven characters of the text will be displayed.
$text = “Enter your text here”;
$substring = substr($text, 0, 7);
echo $substring;
?>
In the following example, the functions “strtoupper” and “strtolower” are respectively used to display uppercase and lowercase characters.
Step 1: A variable is created and assigned a value.
$text = “Enter your text here”;
?>
Step 2: Two new variables are created and the “strtoupper” and “strtolower” functions are added.
$text = “Enter your text here”;
$upper = strtoupper($text);
$lower = strtolower($text);
?>
Step 3: The uppercase and the lowercase version of the text can be displayed by referring to the variables created in step 2 with “echo”.
$text = “Enter your text here”;
$upper = strtoupper($text);
$lower = strtolower($text);
echo $upper;
echo $lower;
?>
Finding a Substring With a Function
The function “strpos” can be used to look for the occurrence of a substring within a string. In other words, “strpos” will look for a specific character or word within a word or sentence.
In this example, “strpos” is used to look for the letter T in a sentence.
Step 1: Create the “strpos” function and add parentheses. The first element placed within the parentheses is the string that will be searched, and the second element is the substring the function will look for. Note that a comma separates the two elements.
strpos(“This is our text” , “t”);
?>
Step 2: Use “if” to display an error message in case the substring could not be found. The function is placed within parentheses and associated with “=== false” to indicate what should be done in case the function is not fulfilled. The next line of code within curly brackets will be executed if the function is false.
strpos(“This is our text” , “t”);
if (strpos(“This is our text” , “a”) ===false); {
echo “The letter could not be found”; }
?>
Doing Math With Functions
PHP has a large number of built-in functions designed to perform arithmetic operations. For instance, the function “round” is commonly used to round number with decimal points. In the following example, “round” is used on a decimal number.
Step 1: A variable is created and the function “round” is associated with it. A decimal number is placed within parentheses so the function can be applied. The following string will cause the number 10 to be displayed.
$roundnumber = round(9.513275578);
echo $roundnumber;
?>
Step 2: It is possible to round a number to a specific decimal by adding a second element to the function. The first element is the number that should be rounded, and the second element indicates at which decimal it should be rounded. In this example, the number 9.513 will be displayed.
$roundnumber = round(9.513275578 , 3);
echo $roundnumber;
?>
Generating Random Numbers With Functions
The function “rand” has already been introduced with the example of the rolling dice. “Rand” needs to be characterized by two elements. Two numbers should be placed between parentheses to indicate the range of numbers the function can use to generate a random number. Note that without the presence of a loop, the function will only generate one number.
In the following example, “rand” is used to generate a random number between 0 and 50.
php
$randomnumber = rand(0 , 50 );
echo $randomnumber;
?>
It is possible to combine several functions. For instance, “rand” can be combined with “strlen” and “substr” to generate a random character from a text.
Step 1: Create a variable with a text and create a second variable that corresponds to the length of the text entered by using “strlen”.
$text = “Enter your text here”;
$length = strlen($text);
?>
Step 2: Create a “rand” function and assign its two elements. The “rand” function is there to generate a random number between 0 and the number of characters of the text minus 1, since 0 refers to the first character.
$text = “Enter your text here”;
$length = strlen($text);
$random = rand(0 , $length Đ 1);
?>
Step 3: Use “substr” to find a substring within a string. The first element placed within parentheses indicates the string the substring should be extracted from. The second element indicates which character needs to be extracted. In this case, the variable $character is used so that a different number is randomly generated every time. The third element indicates the number of characters that should be displayed. It is set to 1, but increasing this number would cause the following characters to appear in order.
$text = "enter your text here";
$length = strlen($text);
$random = rand(0, $length-1);
echo substr($text, $random, 1);
?>
Array Functions
The arrays discussed earlier are actually functions. Functions are very useful when creating lists. “Array” is used to establish a list, “array_push” can be used to add an element to a list, and “count” is used to determine how many elements have been placed in an array.
In the following example, two new customers are added to an array that lists existing customers.
Step 1: Create a variable, associate it with an array, and place elements within the array.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter”);
Step 2: “array_push” is used to add elements to the array created in step 1.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter”);
array_push(“Mary Johnson”);
array_push(“Martin Rodriguez”);
?>
Step 3: The function “count” can be used to keep track of how many elements are placed in an array. After using “array_push” to add two customers to the array, “count” should display the number 5.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter”);
array_push(“Mary Johnson”);
array_push(“Martin Rodriguez”);
echo count($customers);
?>
Organizing Arrays With Functions
The function “sort” is a built-in function that organizes the elements placed in an array. The function “sort” will organize elements in alphabetical or numerical order, while “rsort” will organize elements in reverse order.
In the following example, the array that contains the names of different customers is organized in alphabetical order.
Step 1: Create an array and organize it with “sort”.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter” , “Mary Johnson” , “Martin Rodriguez”);
sort($customers);
?>
Step 2: Display the elements of the array in order by using “echo” and the function “join”. The first element associated with join is the symbol that will be placed between the different elements, and the second element refers to the array that should be joined. In this case, an alphabetical list of the customers will be displayed. Replacing “sort” with “rsort” would cause the names to appear in reverse alphabetical order.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter” , “Mary Johnson” , “Martin Rodriguez” );
sort( $customers );
echo join( “ , “ , $customers) ;
?>
Combining Functions
In the following example, a drawing is organized to randomly select a customer from an array and display their name in uppercase letters.
Step 1: Create an array with the names of the customers and count the number of customers with “count”.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter” , “Mary Johnson” , “Martin Rodriguez” );
$number = count( $customers );
?>
Step 2: Use “rand” to select a random name from the array. The first element of the function should be 0 to refer to the first element of the array, and the second element should be $number -1 since the first element of the array corresponds to 0.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter” , “Mary Johnson” , “Martin Rodriguez” );
$number = count( $customers );
$random = rand( 0, $number-1 );
?>
Step 3: Use “echo” to display the name of the winner. The function “strtoupper” is used to extract a specific element from the array. The array is indicated first and the value between square brackets corresponds to the position of the element that needs to be extracted. In this case, this value is the number generated by “rand”, but it is possible to replace this value by a number to extract the name of a specific customer.
$customers = array( “John Smith” , “Jane Doe” , “Sam Carpenter” , “Mary Johnson” , “Martin Rodriguez”);
$number = count( $customers );
$random = rand( 0, $number-1 );
echo strtoupper( $customers[$random] );
?>
Other Built-In Functions
PHP has many built-in functions but the available functions vary from one system to another. The best way to obtain a comprehensive list of all the built-in functions available is to use phpinfo(). This string of code gives access to information about the server used, predefined functions, and variables and loaded modules.
Step 1: Open a Notepad file and enter this line of code.
The following code can also be used to get a well-organized list of all the functions, but does not include all the details that can be obtained with phpinfo:
function myrow( $id, $data )
{
return "
}
$arr = get_defined_functions();
print_r($arr);
Step 2: Save the file as a php file.
Step 3: Upload this file to a server.
Step 4: Navigate to the webpage that corresponds to the php file. All the information about the server and the PHP module should be there, including a list of built-in functions.
How to Create a Function
Even though there are many built-in functions in PHP, creating a new function is sometimes necessary. In fact, programmers create new functions regularly in order to keep their code as simple as possible. If a block of code is repeated more than once, it is much easier to create a new function instead of using the same block of code. Replacing blocks of code with new functions keeps the code tidy and helps programmers save time when creating code or when going through existing code to make modifications.
It is important to understand function syntax in order to create functions. No matter what the purpose of the new function is, the basic syntax will always remain the same. The syntax used for creating functions is the same as the one used for built-in functions. This is the syntax used to create new functions:
function name( parameters ) {
statement;
}
The keyword “function” is used to create a new function. The name of the function is entirely up to the programmer. It is best to give a function a descriptive name to easily remember its purpose. The parameters, also referred to as arguments, vary in function of the purpose of the function. Parameters can, for instance, be used to describe the calculations that the function will perform. The statement is the line of code that is executed by the function.
Here is an example of a very simple function. This function is named “greeting” and its purpose is to display a welcome message.
Step 1: Create the function “greeting” with a line of code that displays a welcome message.
function greeting() {
echo “welcome guest!”; }
?>
Step 2: Now that the function has been created, it can be used at any time. Adding the function “greeting()” anywhere on this HTML page will cause the line of code to be executed.
function greeting() {
echo “welcome guest!”; }
greeting();
?>
Adding Parameters to a Function
Functions use parameters to perform actions. It is possible to use several parameters as long as they are separated by a comma within the parentheses that follow the name of the function.
In the following example, a function is created to add 2 to a number.
Step 1: The function “plustwo” is created. A parameter is added within parentheses and the statement defines what happens to this parameter, in this case two is added.
function plustwo( $number ) {
echo $number + 2; }
?>
Step 2: Now that the function is created, it can be used. A parameter is defined and placed within the parentheses of the function. In this case, the number 14 will be displayed.
function plustwo( $number ) {
echo $number + 2; }
$example = 12
function plustwo( $example );
?>
VIII. Getting Started With Object-Oriented Programming
PHP makes it possible to create objects. Objects are defined by variables and functions. Variables are referred to as the properties, attributes, or fields of an object, and functions are referred to as the methods of an object. Most of the elements used in PHP are objects, including the arrays and functions discussed earlier.
Objects are used to create bundles of data and function. A template, referred to as a class, is created and used as a model for multiple objects. Each object created from this template or class is called an instance. All the instances are similar to the template to a certain extent, but there are small differences between instances.
For instance, objects are used on message boards. A class of users is created and specific rights such as logging in, posting, and sending private messages are assigned to this class. Every account created is an instance. Another class is created for moderators and different rights are assigned to this class.
How to Create a Class
The function used to create classes is similar to the one used for functions. The keyword “class” is used to indicate that a class is created. A name is chosen for the class and properties are added. This is what the basic syntax for a class looks like: class name{ }
Once a class is created, the keyword “new” can be used to create a new instance. The parentheses would be used to add arguments.
class name{
}
instance1 = new name();
instance2= new name();
instance3= new name();
?>
Adding Properties to a Class
Properties are added within the curly brackets that follow the name of a class. Properties are pieces of information connected to the object. Properties are added by using the keyword “public”.
In the following example, a class is created for customers. Properties include the number of purchases a customer has made, as well as their membership status.
Step 1: Create a class named “customers” and add two properties that correspond to the number of purchases and the membership status.
php
Class customers{
public $purchases
public $status }
?>
Step 2: Set the values of the properties that have been added to the class in the first step. In this example, the class is defined as a group of customers who have made five purchases.
php
Class customers{
public $purchases = 5; }
?>
Step 3: An instance is added to the class with the keyword “new”. It is now possible to see the instance's properties by using the name of the instance followed by -> and by a property.
php
Class customers{
public $purchases = 5; }
$johnsmith = new customers();
echo $johnsmith->purchases;
?>
How to Use Constructors
Objects have a built-in method called a constructor. This method is used to assign properties to a new instance. The constructor method begins with the keyword “construct” and is followed by two underscores.
In the following example, a class of customers is created, and their first and last names, as well as the number of purchases they have made, are added as properties. A constructor is then created so that new instances can be quickly added.
Step 1: Create a class of customers and add three properties.
php
class customer {
public $firstname;
public $lastname;
public $purchases; }
?>
Step 2: A constructor is added to the keyword “construct” and two underscores are used to create a constructor.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases )
}
?>
Step 3: Values are assigned within the constructor. The keyword “$this” refers to the current object.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
?>
Step 4: A method (or function) is added. In this case, the purpose of the method is to greet the customer by name and remind them of how many purchases they have made.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
public function greeting() {
echo "Hello " . $this->firstname . " " . $this->lastname . ". You have made" . $this->purchases . "purchases.";
?>
Step 5: A new instance is added. Now that a constructor has been added and its three properties defined, a new instance can be quickly added by simply listing three values that correspond to the properties within parentheses.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
public function greeting() {
echo "Hello " . $this->firstname . " " . $this->lastname . ". You have made " . $this->purchases . " purchases.";
$johnsmith = new customer( 'John', 'Smith', 5 );
?>
Step 6: A new instance has been added. “$johnsmith” and the function “greeting” can now be used. The last line of code would display the sentence “Hello John Smith. You have made 5 purchases.” Note that there is no need to use $ when referring to a property in a class, which is why “firstname”, “lastname” and “purchases” are written as such.
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
public function greeting() {
echo "Hello " . $this->firstname . " " . $this->lastname . ". You have made " . $this->purchases . " purchases.";
$johnsmith = new customer( 'John', 'Smith', 5 );
echo $johnsmith->greeting();
?>
How to Retrieve Information About an Object in a Class
Built-in methods exist to retrieve information about an instance of a class. For instance, the method “is_a()” can be used to check if an instance belongs to a specific class. The method “property_exist()” allows to check for the existence of a given property associated with an object, and “method_exist()” is used to look for a specific method.
These different methods are followed by parentheses that should include two arguments. The first argument refers to an object, and the second argument can refer to a class, a property, or a method depending on the built-in method used.
In the following example, “is_a()” is used to check if an instance belongs to a class.
Step 1: A new instance is added to the class “customers”.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
$johnsmith = new customer( 'John', 'Smith', 5 );
?>
Step 2: “is_a()” is added to check if $johnsmith belongs to the class “customers”.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
$johnsmith = new customer( 'John', 'Smith', 5 );
is_a($johnsmith, “customer”)
?>
Step 3: “is_a()” is placed within a condition, and a string of code is added to display a message if the condition is fulfilled.
php
class customer {
public $firstname;
public $lastname;
public $purchases;
public function __construct( $firstname, $lastname, $purchases ) {
$this->firstname = $firstname;
$this->lastname = $lastname;
$this->purchases = $purchases;
}
$johnsmith = new customer( 'John', 'Smith', 5 );
if (is_a($johnsmith, “customer”)) {
echo “Customer found”; }
?>
Classes Within Classes
Creating a class within a class means that the sub-class will inherit the properties and methods of the first class. This concept is known as inheritance and is used so that a class can take on most of the properties and methods of another. For instance, a class named “food” could have several sub-classes, including “fruits” and “vegetables”. Both sub-classes can take on most of the properties of the “food” class while remaining separate. The keyword “extends” is used to establish inheritance.
In the following example, a class called “customers” is created and a property establishes free shipping for members of this class. A second class is then created to add more benefits for VIP members.
Step 1: The “customer” class is created and free shipping is added to its properties.
class customer{
public $freeshipping = true;
}
?>
Step 2: A second class is created and inheritance is established thanks to the keyword “extends”.
class customer{
public $freeshipping = true;
}
class vip extends customer {
}
?>
Step 3: A new customer is added to the class “VIP”. This new customer also belongs to the class “customers” thanks to the inheritance relationship between the two classes.
class customer{
public $freeshipping = true;
}
class vip extends customer {
}
$johnsmith = new vip();
?>
Overriding Inheritance
By default, a sub-class will inherit all the properties and methods of the original class. However, it is possible to override some of these properties or methods. If a property or a method is assigned a new value in the code of the sub-class, it will take precedence over the value of the original class.
In the following example, the class “customer” benefits from free shipping and a 20% off discount. The sub-class “VIP” is assigned a new value so its members can benefit from a different discount.
Step 1: Establish a class, a sub-class, and an inheritance relationship.
class customer{
public $freeshipping = true;
public $discount = 20;
}
class vip extends customer {
}
?>
Step 2: The property “$discount” is added to the sub-class, and a new value is created for this property. This new value overrides the one associated with the class “customer”.
class customer{
public $freeshipping = true;
public $discount = 20;
}
class vip extends customer {
public $discount = 50;
}
?>
VII. Going Further With PHP
Flow control, arrays, loops, functions, and object-oriented programming are just the beginning. It is important to become familiar with these key concepts before progressing to more advanced PHP projects. Mastering these different concepts and learning to use them together, for instance, by mixing flow control and loops, or by using functions along with object-oriented programming are great ways to progress.
Practice makes perfect. PHP code does not always work right away. Analyzing the syntax carefully and going back to the examples presented for the different concepts discussed should be useful. Creating PHP code is much easier if one looks for ways to keep things as simple as possible. For instance, creating a new function is preferable to repeating lines of codes. If a PHP code is starting to look confusing, it is time to look for ways to simplify it or to add comments so the code is easier to read and analyze for the programmer.
The best way to practice with PHP is to find a free web server and start creating PHP files. PHP code can also be tested by saving .php files and opening them with a web browser. If the purpose is to eventually create a website, it is best to practice with a web server so one can become familiar with linking pages to other pages, images, or CSS style sheets that already exist on the server.
Challenging oneself is necessary in order to become a better web developer. There are several fun PHP projects that could help a beginner improve their skills, such as creating a very basic blogging platform, a simple message board, a shopping cart, an RSS feed, or a quiz. More advanced users can progress by creating simple PHP based games that rely on random events and statistics.
Beginners might be tempted to start working on PHP code for a website right away. It is best to spend some time on practicing and becoming more familiar with PHP before using this language on a website. PHP code that contains mistakes could prevent the website from being displayed correctly and provide visitors with a poor experience. Besides, low quality PHP code could be a security issue for a website that collects information on users, or sells products.
Learning PHP is an excellent investment of one's time and resources since this language opens up the door to many possibilities when it comes to website development. Mastering PHP makes learning other languages such as C, C++, and C# all much easier.